Webhooks and Google Chat
This post will show you how to create a Go binary that can POST messages to a Google Chat channel, a.k.a Google Space.
Step 1.
Create a channel set up the webhook endpoint. To do this, follow the documentation provided by Google:
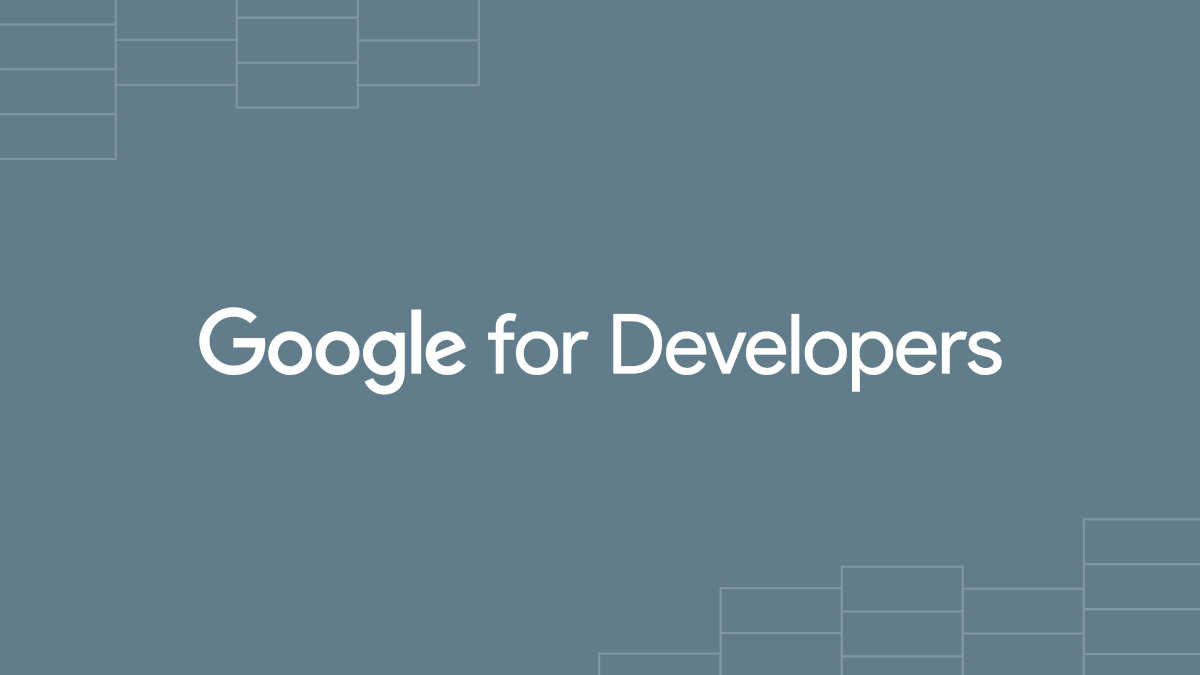
Step 2.
Create the Go application that will send the POST request with your message to the webhook URL.
package main
import (
"bytes"
"encoding/json"
"fmt"
"github.com/joho/godotenv"
"io"
"net/http"
"os"
)
func main() {
if len(os.Args) < 2 {
fmt.Println("Usage: ./go-google-space <message>")
return
}
userMessage := os.Args[1]
client := &http.Client{}
err := godotenv.Load()
if err != nil {
fmt.Println("Error loading .env file")
}
chatSpaceID := os.Getenv("SPACE_ID")
key := os.Getenv("KEY")
token := os.Getenv("TOKEN")
url := fmt.Sprintf("https://chat.googleapis.com/v1/spaces/%s/messages?key=%s&token=%s", chatSpaceID, key, token)
message := map[string]string{"text": userMessage}
messageJson, err := json.Marshal(message)
if err != nil {
fmt.Println("Error marshalling message")
}
req, err := http.NewRequest("POST", url, bytes.NewBuffer(messageJson))
if err != nil {
fmt.Println("Error creating request")
return
}
req.Header.Add("Content-Type", "application/json; charset=UTF-8")
resp, err := client.Do(req)
if err != nil {
fmt.Println("Error sending request")
return
}
defer func(Body io.ReadCloser) {
err := Body.Close()
if err != nil {
fmt.Println("Error closing response body")
}
}(resp.Body)
}
main.go
Compile and run with:
go build -o go-google-space main.go
./go-google-space "Hello from Go!"
Make sure you have the .env
file in the same directory as the binary, containing the values from the generated webhook URL. It should look like this:
SPACE_ID="<SPACEID FROM WEBHOOK URL>"
KEY="<KEY FROM WEBHOOK URL>"
TOKEN="<TOKEN FROM WEBHOOK URL>"
.env file
For more information, check the repository at the link below:
Why did i create this? IDK why.